How Can We Help?
API Documentation
The eSchedule API allows organizations the ability to connect to our RESTful service. If authenticated, HTTP GET requests can be made. The endpoints return a JSON object containing eSchedule information.
Please contact our Support Team to obtain your token.
Function: read
This endpoint returns a JSON object containing schedule/shift data, depending on the date range provided in the request. By default, if no parameters are provided, the data returned will be ALL of today/tomorrows shifts. A max date range of 30 days is allowed. Currently the only view other than all is ‘Prime Shifts’.
If connected properly, the shift data will be returned with a root of ‘Shifts’, and a root of ‘Error’ if there’s an issue.
URL: https://yourAgency.myesched.com/eschedule_api/product/read.php?
Headers
token | your_token (contact support to get this) |
Params
start_time | 9/10/2018 |
end_time | 9/16/2018 |
view | prime_shifts |
The shift data will be key-valued pairs formatted as so:
{
“first_name”: “John”,
“last_name”: “Doe”,
“shift_date”: “09/10/2018”,
“shift_index”: “10”,
“position_index”: “1”,
“shift_name”: “Day Float 1”,
“position”: “Officer”,
“emt_level_code”: “P”,
“observer”: null,
“start_time”: “2018-09-10T10:00Z”,
“end_time”: “2018-09-10T22:00Z”,
“shift_notes”: “Sample Notes”
}
Note: Returned datetimes are returned in TZ format (UTC), while the shift_date will be returned as the agencies local time
Example Code
Language cURL
curl –request GET \
–url ‘https://yourDomain.myesched.com/eschedule_api/product/read.php?start_time=9/10/2018&end_time=9/16/2018’ \
–header ‘token: some_value’
—————————————————————————————————————————————-
Language jQuery
var settings = {
“async”: true,
“crossDomain”: true,
“url”: “https://yourAgency.myesched.com/eschedule_api/product/read.php?start_time=9/10/2018&end_time=9/16/2018”,
“method”: “GET”,
“headers”: {
“token”: “some_value”
}
}
$.ajax(settings).done(function (response) {
console.log(response);
});
—————————————————————————————————————————————-
Language Python
import requests
url = “https://yourDomain.myesched.com/eschedule_api/product/read.php”
querystring = {“start_time”:”9/10/2018″,”end_time”:”9/16/2018″}
headers = {‘token’: ‘some_value’}
response = requests.request(“GET”, url, headers=headers, params=querystring)
print(response.text)
Language Node
var http = require(“https”);
var options = {
“method”: “GET”,
“hostname”: [
“yourDomain”,
“myesched”,
“com”
],
“path”: [
“eschedule_api”,
“product”,
“read.php”
],
“headers”: {
“token”: “some_value”
}
};
var req = http.request(options, function (res) {
var chunks = [];
res.on(“data”, function (chunk) {
chunks.push(chunk);
});
res.on(“end”, function () {
var body = Buffer.concat(chunks);
console.log(body.toString());
});
});
req.end();
Function: clockin
This function returns a JSON object containing either a ‘success’ or ‘error’ array. Parameters vary based on when/what the user is clocking in for. Token and User_id are always required.
URL: https://yourAgency.myesched.com/eschedule_api/product/clockin/index.php?
Headers
token | your_token (contact support to get this) |
Params
User_id | User.name |
Comments Required if clocking in to scheduled shift outside of grace period, and for All Activities | “Clocking in” |
time_clock_activities_id Required if not scheduled. Must be from valid Time Clock Activity | “19” |
Other_activity Comment for Other Activity – Required if Activity=Other | “Off-duty response” |
Successful Clockin returns:
{“success”:”user.id has been clocked in”}
Error codes
Error 100 – Invalid User ID or Ambulance ID
Error 101 – Invalid User ID
Error 200 – Invalid activities ID – Also returns array of valid time_clock_activities_id (See below)
Error 201 – Other Activity Description Required
Error 202 – Not on schedule, Returns valid time clock activities ids – – Also returns array of valid time_clock_activities_id
Example json:
{“tca”: [
{
“time_clock_activities_id”: “5”,
“description”: “Other”
},
{
“time_clock_activities_id”: “2”,
“description”: “Training”
}
],
“error”: {
“code”: “202”,
“message”: “Not on schedule, time clock activity required”
}
}
Output – Error 300 – Clockin for Activity – Comments Required
Output – Error 301 – In Early – Comments Required
Output – Error 302 – In Late – Comments Required
Output – Error 401 – User already clocked in
Ex JSON for errors: {“error”:{“code”:”301″,”message”:” In early or in late – Comments Required “}}
Function: clockout
This function returns a JSON object containing either a ‘success’ or ‘error’ array. Token and User_id are always required. Comments are required if clocking out of scheduled shift outside of grace period
URL: https://yourAgency.myesched.com/eschedule_api/product/clockout/index.php?
Headers
token | your_token (contact support to get this) |
Params
User_id | User.name |
Comments Required if clocking out of scheduled shift outside of grace period | “Clocking out” |
Successful Clockout returns:
Json: {“success”:”user.id has been clocked out”}
Error codes
Error 100 – Invalid User ID or Ambulance ID
Error 101 – Invalid User ID
Error 303 – Out early – Comments Required
Error 304 – Out late – Comments Required
Json: {“error”:{“code”:”304″,”message”: “Out Late – Comments Required “}}
Output – Error 400 – User is not clocked in
Json: {“error”:{“code”:”302″,”message”: “User is not clocked in”}}
Function: onShift
This function returns a JSON array of all people currently on shift
URL: https://yourAgency.myesched.com/eschedule_api/product/onshift/index.php?
Headers
Token your_token
Output
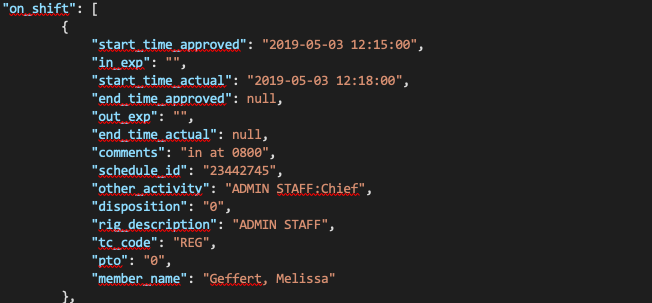
Function: add_profile
This endpoint adds a new user to eSchedule.
URL: https://yourAgency.myesched.com/eschedule_api/eschedule_api/product/profile/add_profile.asp
Headers
token | your_token (contact support to get this) |
logonuid | An active administrator user id. It is recommended you create a separate administrator account for API’s that require an administrator logon. |
logonpw | Administrators password |
Params
column_name | Required | type | length | Notes |
user_id | Y | character | 50 | Minimum length of 4. User Id can only include numbers, letters or a period. Fingerprint reader customers: max length 45 minus super-secret password length. |
password | Y | character | 50 | minimum length of 4 |
last_name | Y | character | 50 | |
first_name | Y | character | 50 | |
user_member_type | Y | character | Valid values: Compensated, Volunteer | |
startdate | Y | date | date | |
middle_initial | character | 4 | ||
sex | character | 6 | Valid values: Male, Female | |
user_career_type | character | Valid values: Full Time, Part Time, Per Diem | ||
birthdate | date | |||
character | 100 | Valid email address | ||
text_message_address | character | 50 | Valid email address | |
emt_level | character | Valid EMT Levels based on your site configuration | ||
clearances | character | unlimited | Comma delimited list of positions the EE is cleared for. Validated based on your site configuration. | |
title | character | 50 | ||
ssn | character | 4 | ||
memberid | character | 50 | ||
clock_number | character | 15 | ||
emt_num | character | 12 | ||
address | character | 50 | ||
address2 | character | 50 | ||
city | character | 50 | ||
state | character | 50 | ||
zip | character | 50 | ||
home_phone | character | 20 | ||
work_phone | character | 20 | ||
cell_phone | character | 20 | ||
station_phone | character | 50 | ||
emergency_contact | character | 50 | ||
emergency_contact_phone | character | 20 | ||
employer | character | 50 |
Return Codes
201 Created – User Added
400 Bad Request – Missing User Id or Password (logonuid or logonpw)
400 Bad Request – Data did not pass edit checks. Return message will describe invalid data.
400 Bad Request – Exceeded maximum active users, contact eSchedule support.
400 Bad Request – user_id already exists
401 Unauthorized – Missing Token
401 Unauthorized – Missing Token
401 Unauthorized – setSessionVarsWithPW failed. Status =
1001 = Invalid Ambulance ID
1002 = Invalid Logon ID
1003 = Inactive Logon User
1004 = Invalid Logon Password
403 Forbidden – Invalid Token
403 Forbidden – logonuid not authorized for this function
Function: get_absent_and_late_punches
This returns a json of absent and late data from eSchedule. The json will match the data found in the eSchedule Absentee and Late Report found under the Time Clock menu (for Time Clock Administrators).
URL: https://yourAgency.myesched.com/eschedule_api/product/get_absent_and_late_punches/default.asp?
Headers
token | your_token (contact support to get this) |
logonuid | An active administrator user id. It is recommended you create a separate administrator account for API’s that require an administrator logon. |
logonpw | Administrators password |
Params
column_name | No | Notes |
from_date | No | defaults to today |
to_date | No | defaults to today plus 1 month |
user_id | No | Filters the list to a single user. Must be a valid user id, otherwise returns “No punches found for this request.” |
user_member_type_id | No | defaults to 1 – Compensated, valid values 1 – Compensated, 2 – Volunteer, All |
status_id | No | defaults to 1 – Active (users), valid values 1 – Active, 2 Inactive, ALL |
user_dept_id | No | defaults to All, must be a valid dept ID (numeric) otherwise returns “No punches found for this request.” Contact eSchedule support for a list of departments and their associated user_dept_id. |
The profile data will be key-valued pairs formatted as so:
{
“start_time_approved”: ”1/3/2023 5:00:00 AM”,
“end_time_approved”: ”1/3/2023 1:20:00 PM”,
“start_time_actual”: ”1/3/2023 8:15:00 AM”,
“end_time_actual”: ”1/3/2023 1:51:00 PM”,
“comments”: ”Large accident on the way to work”,
“time_clock_id”: ”123456789″,
“other_activity”: ””,
“schedule_id”: ”987654321″,
“num”: ”45″,
“description”: ”In Late”,
“code”: ”IL”,
“member_name”: ”Doe, Jon”,
“last_name”: ”Doe”,
“first_name”: ”Jon”,
“schedule_start_time”: ”1/3/2023 7:30:00 AM”,
“schedule_end_time”: ”1/3/2023 4:00:00 PM”,
“position_description”: ”Medic”,
“rig_description”: ”Station 1″,
“time_clock_activities_description”: null,
“pay_type”: ”Regular”,
“user_id”: ”jon.doe”
}
Return Codes
200 OK – membership data returned
401 Unauthorized – Missing Token
400 Bad Request – Missing User ID or Password (logonuid or logonpw)
400 Bad Request – No members found for this request.
400 Bad Request – (with one or more of the following messages)
from_date is invalid.
to_date is invalid.
user_member_type_id is invalid.
status_id is invalid.
user_dept_id is invalid.
403 Forbidden – Invalid Token
403 Forbidden – Invalid Ambulance ID Code 1001
403 Forbidden – Invalid User ID Code 1002
403 Forbidden – Inactive User Code 1003
403 Forbidden – Invalid Password Code 1004
403 Forbidden – logonuid not authorized for this function.
Function: get_profile_data
This returns a json of membership data from eSchedule. The json will match the data found in the eSchedule Member Report found under the Administration menu.
URL: https://yourAgency.myesched.com/eschedule_api/eschedule_api/product/profile/get_profile_data.asp
Headers
token | your_token (contact support to get this) |
logonuid | An active administrator user id. It is recommended you create a separate administrator account for API’s that require an administrator logon. |
logonpw | Administrators password |
Params
column_name | Required | notes |
user_id | No | Valid User ID, filters list to a single member. See User Management under Administration for the user ids in your site. |
The profile data will be key-valued pairs formatted as so:
{
“user_id”: ”jon.doe”,
“Last Name”: ”Doe”,
“First Name”: ”Jon”,
“MI”: ””,
“Address”: ””,
“Address Line 2″: ””,
“City”: ””,
“State”: ””,
“Zip”: ””,
“sex”: ””,
“Last 4 of SSN”: ””,
“Title”: ””,
“Member ID”: ””,
“Home Phone”: ””,
“Work Phone”: ””,
“Cell Phone”: ””,
“Pager”: ””,
“Station Phone”: ””,
“Emergency Contact Name”: ””,
“Emergency Contact Phone”: ””,
“Email”: ”jon.doe@yahoo.com”,
“Text Message Address”: ””,
“Employer”: ””,
“User Type”: ”Basic”,
“Status”: ”Active”,
“Separation Reason”: ””,
“Last Logon Date”: ”3/27/2023 10:46:00 AM”,
“Birthday”: ”11/19/1991″,
“Start Date”: ”11/29/2021″,
“Separation Date”: ””,
“Years of Service”: ”1 years, 10 months”,
“Clock Number”: ”919″,
“Member Type”: ”Compensated”,
“Career Type”: ”Full Time”,
“Beginning Service Hours”: ”0″,
“EMT Level”: ”EMT Basic”,
“EMT Num”: ””,
“Separation Description”: ””,
Dept and Division are optionally returned only if configured in your site
“Dept”: ”Shoreline”,
“Division”: ”AmbuServe”,
User Defined Data is optionally returned only if configured in your site
“Cleared Date”: ”1/1/2021″,
Certification Data is optionally returned only if configured in your site
“CPR - Exp Date”: ”12/2/2023″,
“CPR - Cert Num”: ””,
“CPR - Issued Date”: ”12/2/2021″
}
Return Codes
200 OK – membership data returned
401 Unauthorized – Missing Token
400 Bad Request – Missing User ID or Password (logonuid or logonpw)
400 Bad Request – No members found for this request.
403 Forbidden – Invalid Token
403 Forbidden – Invalid Ambulance ID Code 1001
403 Forbidden – Invalid User ID Code 1002
403 Forbidden – Inactive User Code 1003
403 Forbidden – Invalid Password Code 1004
403 Forbidden – logonuid not authorized for this function.
Function: get_absent_and_late_punches
This returns a json of punch data from eSchedule. The json will match the data found in the eSchedule Punch Report found under the Time Clock menu (for Time Clock Administrators).
URL: https://yourAgency.myesched.com/eschedule_api/product/get_punches/index.php?
Headers
token | your_token (contact support to get this) |
Params
column_name | Required | Notes |
user_id | No | Filters the list to a single user. Must be a valid user id, otherwise returns “No punches found” |
user_member_type_id | No | defaults to ALL: Valid values 1 = Compensated, 2 = Volunteer, ALL |
from_date | No | defaults to today |
to_date | No | defaults to today plus 1 month |
status_id | No | defaults to 1 – Active (users), valid values 1 – Active, 2 Inactive |
division_id | No | defaults to ALL, must be a valid division ID (numeric) otherwise returns “No punches found” Contact eSchedule support for a list of division IDs. |
break_info | No | Adds break information (if found) to the JSON. Valid Values – True. If this parameter is not sent or is not True, break information will not be sent. |
The profile data will be key-valued pairs formatted as so:
{
“punches”: [
{
“user_id”: ”jon.doe”,
“last_name”: ”Doe”,
“first_name”: ”Jon”,
“clock_number”: ”0001″,
“start_time_approved”: ”2023-09-15 09:05:00″,
“end_time_approved”: ”2023-09-15 17:13:00″,
“pay_type_description”: ”Regular”,
“schedule_start_time”: ”2023-09-15 08:30:00″,
“schedule_end_time”: ”2023-09-15 17:00:00″,
“disposition”: ”1″,
“schedule_id”: ”43431537″,
“time_clock_activities_id”: null,
“time_clock_id”: ”10047528″,
“comments”: ”In: in Out: OUT Total Minutes on break : 170 minutes”,
“activity”: ”Billing: Billing Manager”,
“other_activity”: ””,
“pto”: ”0″,
“rec_type”: ”schedule”,
“last_updated_name”: ”Jane Smith”,
“last_update_date_time”: ”2023-09-25 09:55:39.000″,
“breaks”: [
{
“break_type”: ”Lunch”,
“break_start_time”: ”2023-09-15 11:37:00″,
“break_end_time”: ”2023-09-15 13:57:00″
},
{
“break_type”: ”Lunch”,
“break_start_time”: ”2023-09-15 17:54:00″,
“break_end_time”: ”2023-09-15 18:24:00″
}
]
}
]
}
Return Codes
200 OK – punch data returned
No Token Provided
No Punches Found